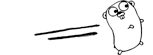
See, normally reviewers concetrate on what they didn't like in a language, but I think that's way too easy! The things we don't like are most probably the things we aren't accustomed with, and with some experience they wouldn't annoy us any more. So don't gripe, just be easy on Go!
As I learned from the book, Plan 9 project*, the (supposed to be) successor of Unix, included a language called Limbo which was a lot like Go already, which built on an earlier langauge called Newsqueak. So surprisingly, there's a lot of history showing up here!
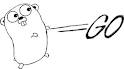
So let's start with:
1. multiple assignement
...a la Python:
x, y := 11, 12 _, y := 22, 23
As you can see the semicolons are not needed too - gives you a nice Python / F# / Clojure feeling!
If you have mutliple assignament, you get multiple return values for free, as in the following function**:
func Write(file *File, b []byte)(int, Error)See? You just specify the returns in parens after the parameters. And that leads us to the next goodie connected to the multiple assignment:
1a. the ok-idiom
it goes like that:
if ok, x = foobar(y); ok {
// do sth
...
}
So no more if(foo() == -1)! Instead you can write: if nr, err = write(f, buf); err == nil {
// do sth
...
}
You can assign and test a value inside of the "if "clause. Additionally the parens can be omitted too with "if" - more Python / F# feeling! Nice, isnt it?2. named return parameters
We can assign names the return parameters:
func write(file *File, b []byte)(n int, err Error){ ... return }When we name the returns, the compiler will initialize them with defaults (i.e. their zero-values)! We can eithet refer to them inside of the function (!!) or even omit them from the return statement, and then they will be used automatically with the initialized values. One thing less to worry about.
3. maps (and strings)
They are part of the language and not in the library. I wish they'll be that way in C++ too. Come on, D has done that!. Add this to the {} initalization syntax and you can write (almost LISP, Python or Perl style)***:
var opmap = map[int]string{ ADD: ”+”, SUB: ”-”, MUL: ”*”, DIV: ”/”, }Well, speaking frankly, the {} initialization is possible in C++ too, alas only as of C++11.
4. defer for destructors
You can define Deferred actions, i.e. actions which are executed on the end of scope only:
file.Open(”filename.in”) defer file.Close()It's a nice replacement for destructors (see my previous post here). And it looks better that some "using" constructs from other languages.
5. duck typing
Go has duck typing, but it's not C++ type of template duck typing. You don't have to mention a specific interface (yes, Go has a notion of interfaces!), it suffices to implement them ***:
type I interface { Get() int Put(int) }And then you just implement the required functions for a data type (see note ** below), and it will automaticall comply with the interface I:
type S struct { i int } func (p *S) Get() int { return p.i } func (p *S) Put(v int) { p.i = v }like that:
func f(p I) { p.Put(1) } var s S f(&s)OK, so whats the difference to C++ type duck typing? C++ doesn't care about types: a template with two parameters simply takes two things, and then tests if they have the methods the template code requires:
template <type T> void foo(T& t) { if(t.canBark()) t.bark(); }How's that different from Go? C++ gets problems with overloading in that context: it sees only a function that takes 1 thing. A totally generic thing! So you cannot define a second template with the same name and a different argument type, because at the definition level there aren't types, only things! That's why there's no range or complete container overloads for SLT algorithms (see H.Sutter's blogpost).
6. generic switch
Just like Groovy, Go has a very flexible switch statement:
switch c { case ’ ’, ’?’, ’&’, ’=’, ’#’, ’+’: return true case c < 'a': fallthrough case c > 'A': return true default: return false }I mean, ever language needs something like that: case switch for strings and other types! And did you notice that fallthrogh must be stated explicitly? I like that.
7. the go keyword.
I liked the idea of introducing the word "go" as keyword to start a parallel "goroutine":
int i go foobar(i)I just find it funny :), that's all...
Note:
I admit, I didn't describe the features that make Go to stand out, only a couple of those which particularly appealed to me. For more info on: function definitions, val notation, control structures, function literals (i.e. lambdas), goroutines, etc please read the book by yourself!
---
* a note aside: as pointed out several times on the web, there's a notable similarity between the logos of Plan 9 and Go. BTW: don't you think they are cute?
** more precise, you'd rather define a function like this:
func (file *File) Write(b []byte)(int, Error)i.e. a function working on the File type, and invoke it like:
nr, err = f.Write(buf)But this is the part of the OO stuff so I skipped it in this post.
*** code example from the "Learning Go" book.